Today, I messed around with the unless keyword in Ruby. It’s kinda like the opposite of if, you know? I figured it’d be cool to try it out and see how it works in a real-world scenario, even though it’s pretty simple.
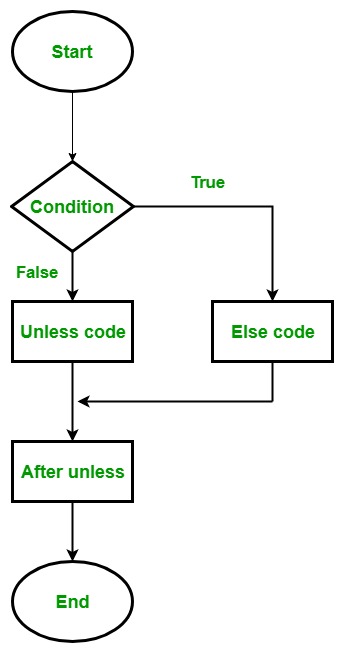
So, I started by opening up my trusty terminal and firing up IRB, the interactive Ruby shell. That’s where I like to test stuff out, just to get a feel for it before putting it into a bigger program.
Experimenting with Unless
First, I defined a simple variable.
my_number = 10
Then, I used an unless statement. The basic idea is that the code inside the unless block only runs if the condition is false.
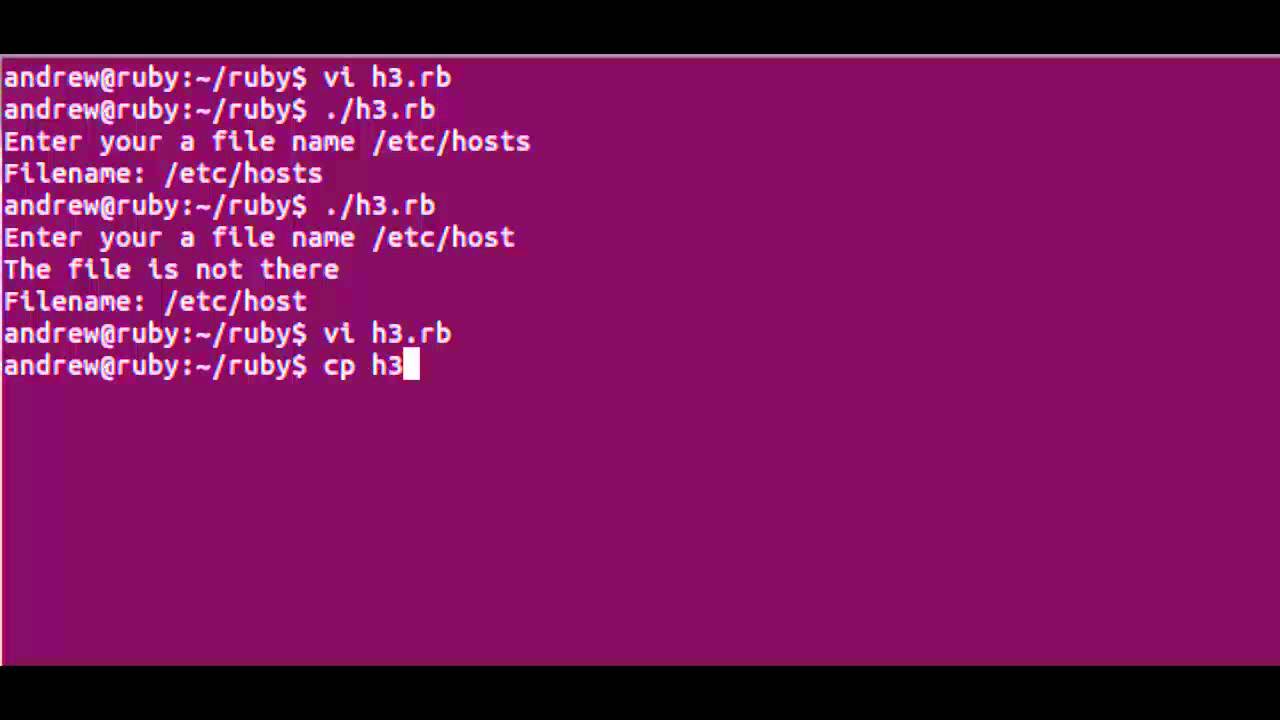
unless my_number < 5
puts "The number is NOT less than 5"
end
I ran that, and sure enough, it printed “The number is NOT less than 5” to the console. Because, well, 10 isn’t less than 5. The condition was false, so the code inside the unless block executed.
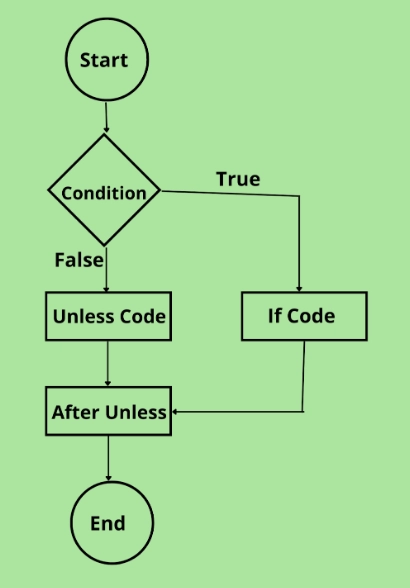
Then, I thought, what if I change the condition? I tried this:
unless my_number > 20
puts "The number is NOT greater than 20"
end
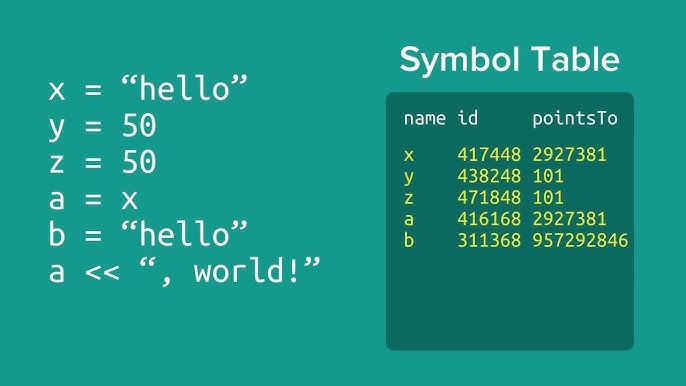
This time, the condition (my_number > 20) is also false, since 10 is not greater than 20, So it printed, “The number is NOT greater than 20.”
Making it a Little More “Real”
Okay, that was all pretty basic. I wanted to do something a little more practical. So I thought, I’ll simulate checking if a user is logged in.
First create a variable.
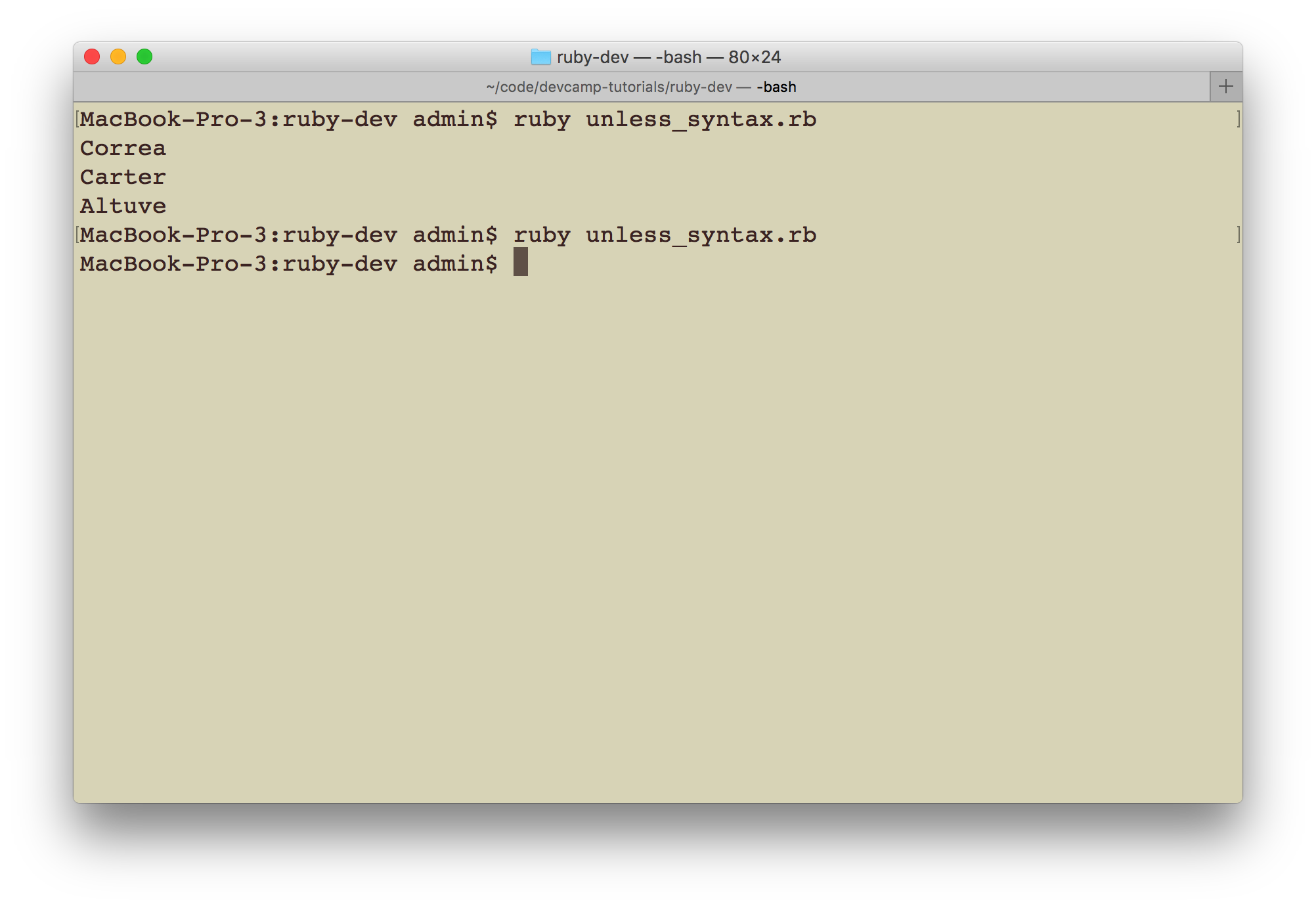
is_logged_in = false
Then use my unless:
unless is_logged_in
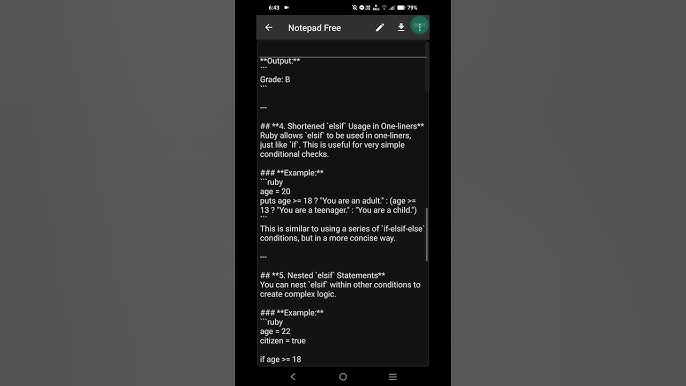
puts "You need to log in!"
end
Since is_logged_in was false, the “You need to log in!” message popped up. Perfect. If I set is_logged_in = true
, that message wouldn’t show, because the condition in the unless would be true, so it won’t execute.
Doing Stuff Inline
Ruby lets you do these one-liner unless statements, too. I gave that a shot:
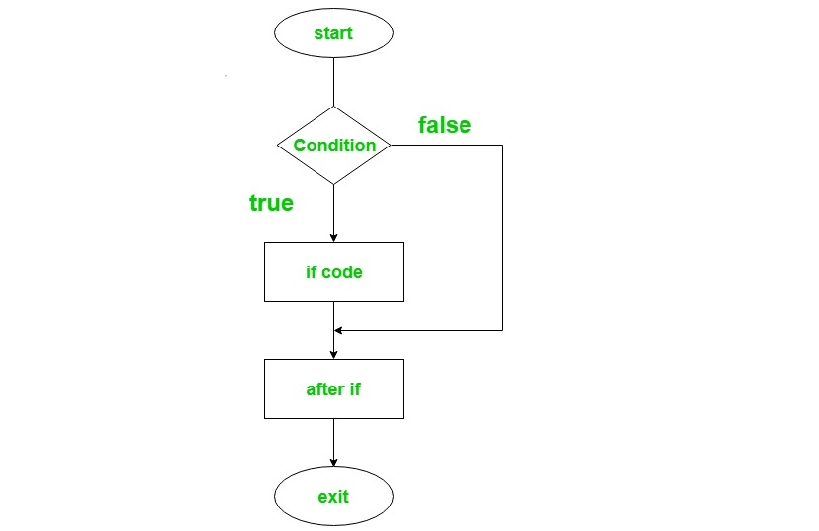
puts "Welcome, user!" unless is_logged_in
Since I lefted is_logged_in = false
from before, it did show up. But if is_logged_in = true
it won’t show anything. Same logic, just shorter.
My wrap up
So, that’s my little adventure with unless in Ruby. It’s not rocket science, but it’s another tool in the toolbox. I can see how it might make code a bit cleaner in some situations, especially when you’re dealing with negative conditions. It’s all about readability, you know? It is my personal experience!