Okay, let me tell you about this thing I worked on recently, this “gs snowflake” idea.
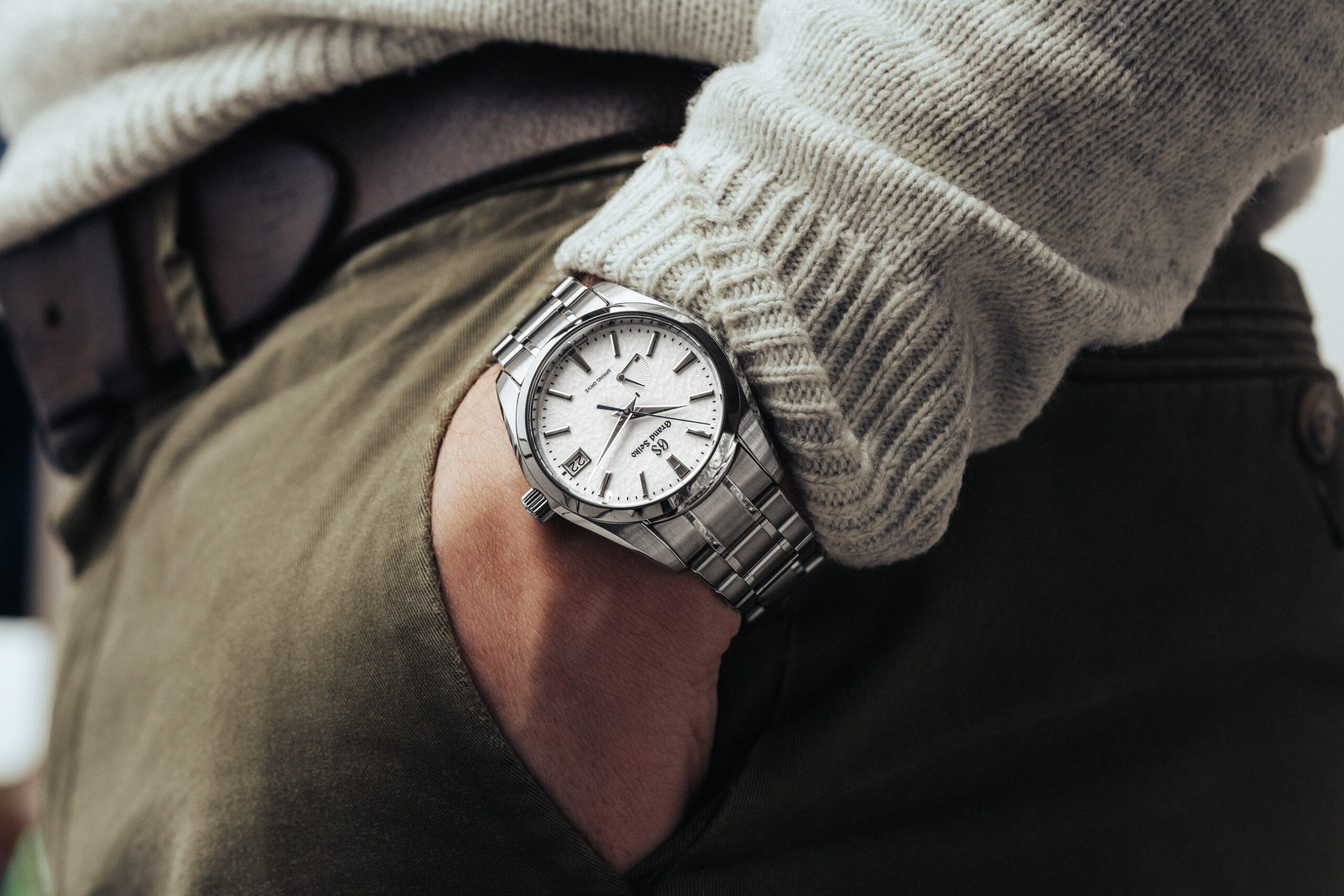
The Problem We Had
So, we started hitting this annoying snag. We’ve got services popping up left and right, written mostly in Go. Great, right? Fast, easy to deploy. But then came the IDs. We needed unique IDs for everything – users, orders, random records. Simple database auto-increments weren’t cutting it anymore. Things got complicated when data came from different places, or when we needed an ID before saving to the database. Plus, trying to coordinate sequences across different machines? Forget it. Total mess waiting to happen.
Looking for a Fix
I remembered reading about how Twitter solved this with something called Snowflake. Time-based, machine ID, sequence number – sounded pretty solid for distributed stuff. So, I started digging around for a Go version. Found a bunch of libraries on GitHub, some looked way too complex, others barely maintained. I settled on one that seemed reasonably straightforward. Let’s just call it the “gs snowflake” package for now, doesn’t matter which specific one it was.
Getting It Working
Pulling the library in was easy, just a standard `go get`. The basic usage looked simple enough: create a generator thingy, call a method, boom, you get an ID. The tricky part, and the part that always trips people up with Snowflake, is the worker ID (or machine ID, whatever you want to call it).
This part was crucial. Each running instance of our service needed a unique worker ID. If two instances had the same ID, they could generate the same Snowflake ID at the same millisecond. Bad news. How do you assign these unique IDs automatically when services scale up and down?
- First idea: Hardcode them? Nope, terrible idea.
- Second idea: Use environment variables? Better. We could inject a unique ID when starting each container or instance.
- Third idea: Maybe some kind of coordination service? Like grab an ID from Consul or etcd on startup? Seemed like overkill for us at the time.
We went with the environment variable route. Seemed like the simplest thing that could possibly work. Wrote a small wrapper around the “gs snowflake” library initialization. It would read `WORKER_ID` from the environment. If it wasn’t there or wasn’t a valid number in the allowed range, the service would just refuse to start. Better to fail loudly than generate duplicate IDs silently, right?
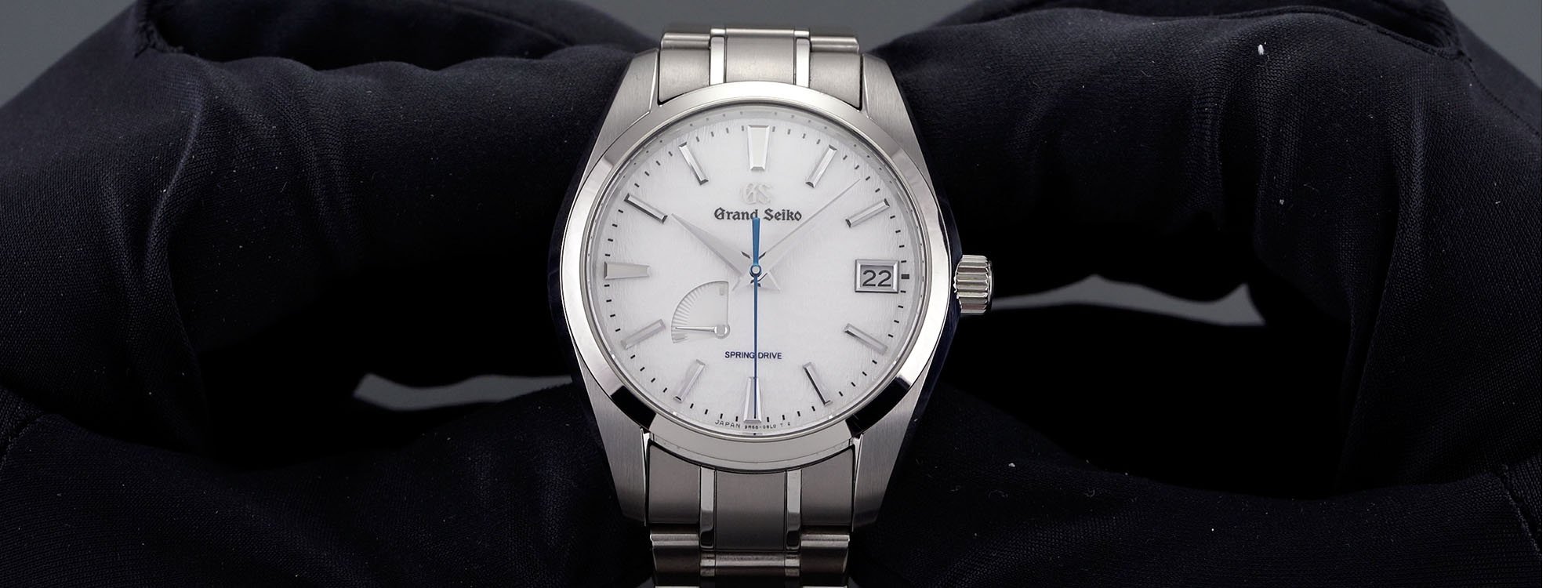
Setting this up took a bit of fiddling with our deployment scripts to make sure each pod or instance actually got a unique number passed in. Had a few deployment failures initially because the script logic wasn’t quite right, or the range of allowed IDs in the library was smaller than we thought. We had to check the library’s code to see how many bits it used for the worker ID.
Testing and Rollout
Once we got the initialization sorted, I ran some local tests. Just hammered the generator in a loop, printed out a million IDs, checked they were increasing mostly monotonically (they should, because of the timestamp part), and made sure I didn’t see obvious duplicates. Looked okay.
Then we rolled it out to a less critical internal service first. Watched the logs, checked the generated IDs in the database. Everything seemed fine. No collisions reported, performance was good – generating an ID is super fast anyway.
Final Thoughts
So yeah, this “gs snowflake” approach worked out for us. It solved the unique ID problem without needing constant database roundtrips or complex coordination. It’s pretty simple conceptually.
The main headache is still managing those worker IDs. You absolutely need a reliable way to assign unique ones to every running instance. The environment variable method works for our current setup, but I can see it getting messy if we had thousands of instances or different deployment methods. You gotta be disciplined about it.
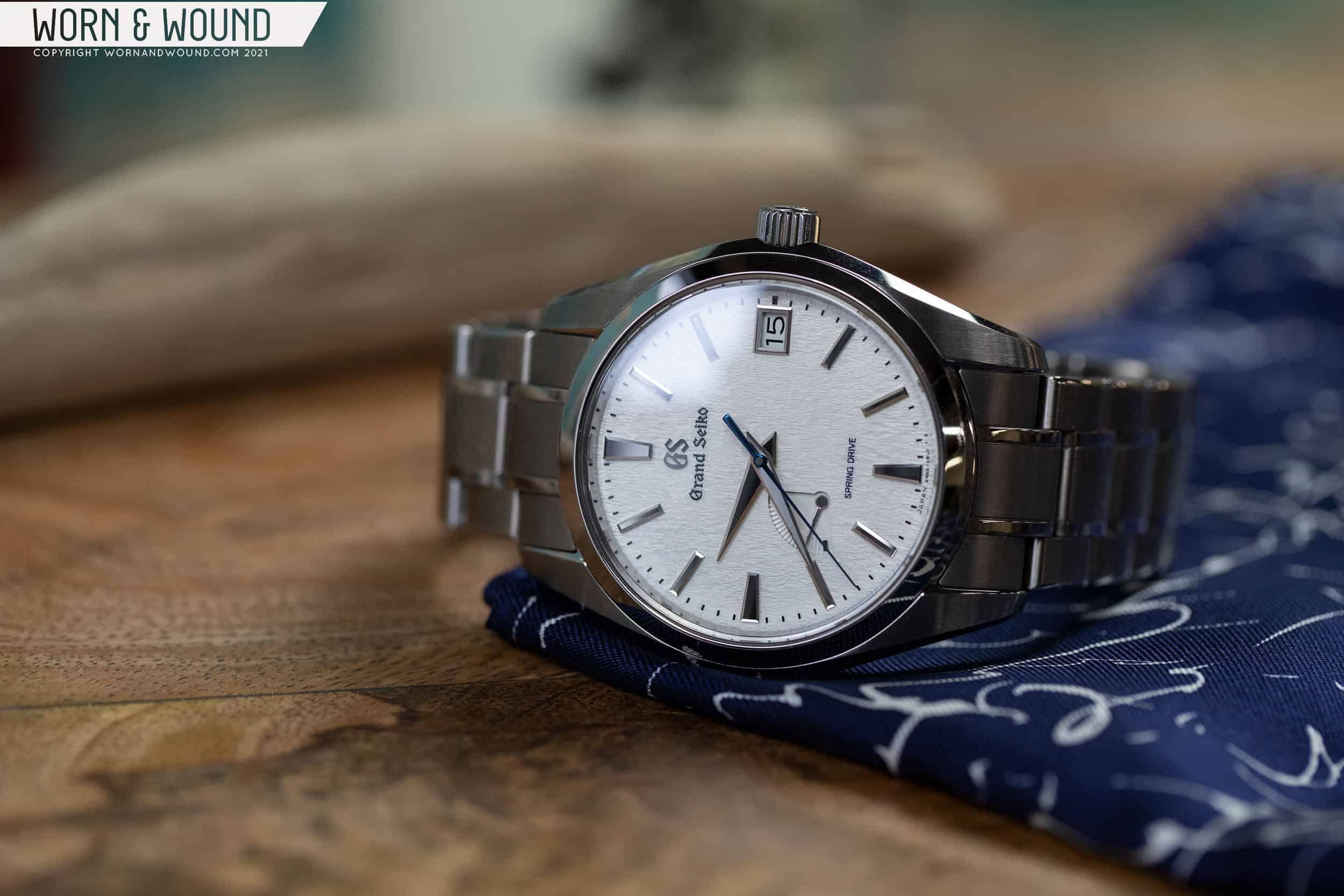
Overall, it was a practical solution to a common problem in distributed systems. Didn’t require rewriting the universe, just carefully plugging in a well-understood pattern. Happy with how it turned out, despite the initial fiddling to get the worker IDs right.