Alright, let’s talk about this haversack 12797 thing. I messed around with it yesterday, and here’s how it went down.
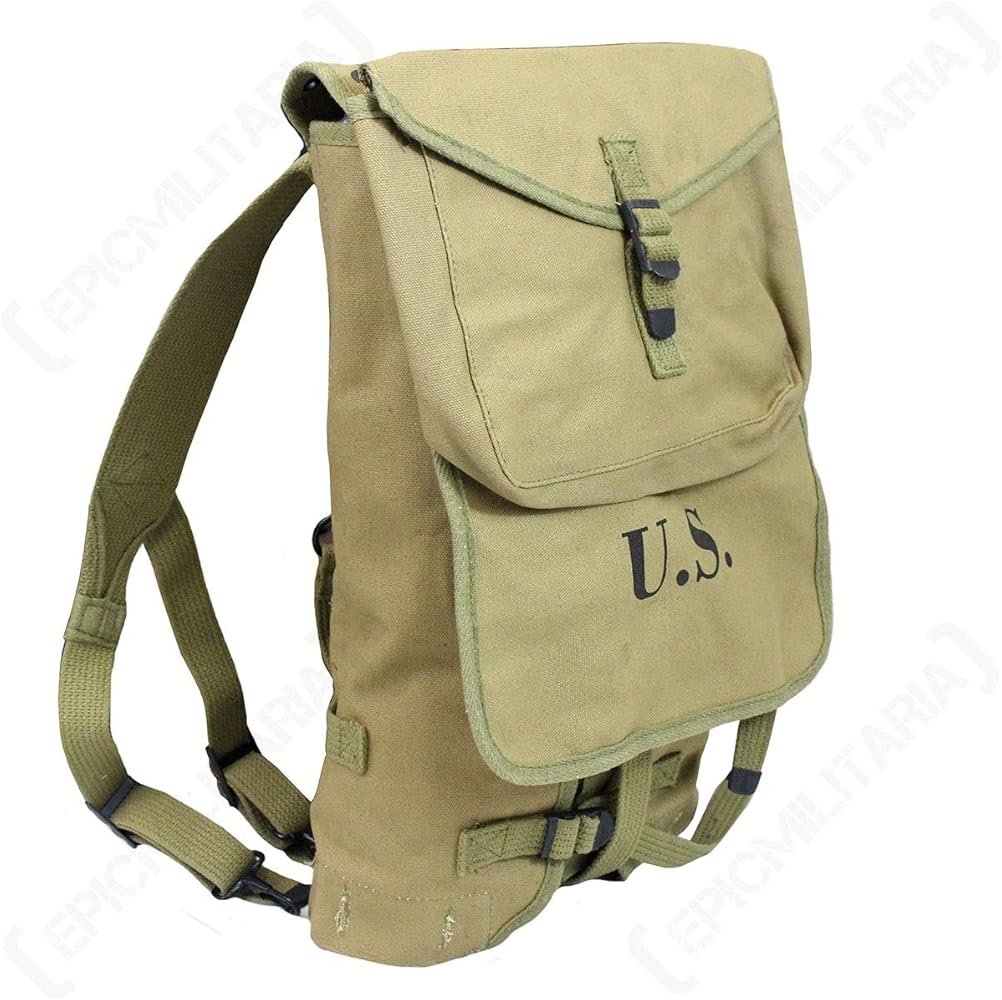
First off, I stumbled upon this problem online, can’t remember exactly where, but it looked interesting enough to give it a shot. The description was kinda vague at first glance, something about packing items into a knapsack with limited capacity, typical knapsack stuff, right? But then there were these weird constraints or conditions, haversack 12797. That threw me off for a bit.
So, I started by trying to really understand what the problem was asking. I grabbed a pen and paper – yeah, old school – and started jotting down notes. What are the inputs? What’s the output supposed to be? What are the rules? After a few read-throughs, and a lot of scratching my head, I think I finally got a handle on it.
Next up, I thought about the brute-force approach. You know, try every single combination of items and see if it fits within the capacity. But I quickly realized that was gonna be way too slow. There were too many items, and the time complexity would be through the roof. So, I ditched that idea pretty quick.
Then, I remembered the classic dynamic programming solution for the knapsack problem. That seemed like a more promising approach. I started sketching out the DP table, thinking about the states and transitions. The state would probably be something like `dp[i][w]`, where `i` is the number of items considered so far, and `w` is the current weight in the knapsack. The value of `dp[i][w]` would be the maximum value we can get with these `i` items and weight `w`. Makes sense, right?
Okay, so the base case would be `dp[0][w] = 0` for all `w`, meaning if we have no items, the value is zero. Then, the transition would be something like: either we include the `i`-th item or we don’t. If we include it, then the value would be `dp[i-1][w – weight[i]] + value[i]`, and if we don’t include it, then the value would be `dp[i-1][w]`. We take the maximum of these two values.
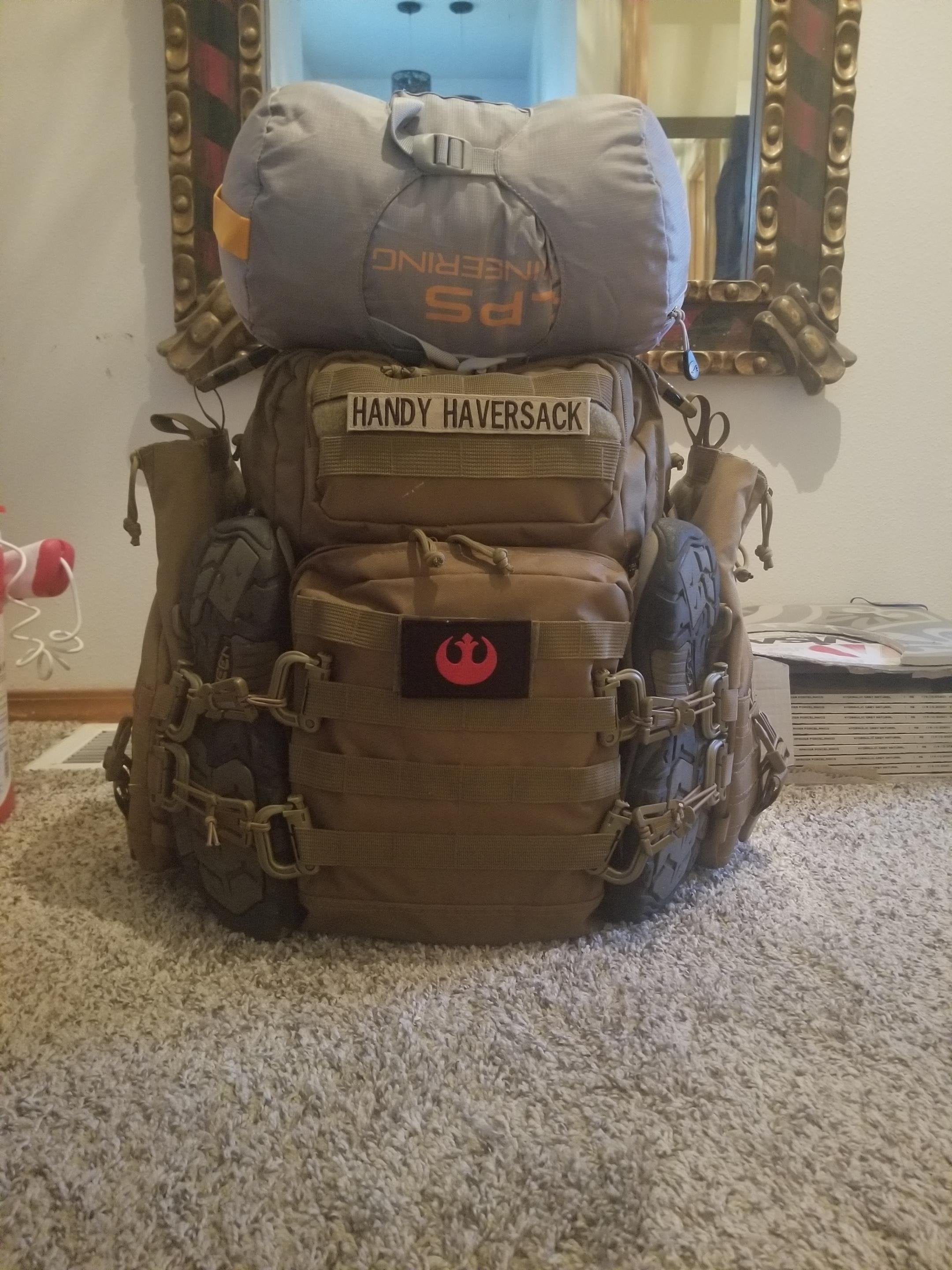
I started coding up this DP solution in my favorite language, Python. I set up the DP table, initialized the base case, and then looped through the items and weights, filling in the table according to the transition rules. I had to be super careful about the indexing, making sure I wasn’t going out of bounds. Debugging DP code can be a real pain!
I ran the code with a few test cases, and it seemed to be working…ish. It was giving the right answers for some cases, but wrong answers for others. Ugh! So, I started debugging. I printed out the DP table after each iteration to see what was going on. I noticed that some of the values were not being updated correctly. I had a subtle bug in my transition logic. After staring at the code for a while, I finally found it and fixed it. It was a simple off-by-one error, but it was causing all sorts of problems.
After fixing that bug, the code started passing all the test cases. Yay! But I wasn’t quite done yet. I wanted to make sure the code was efficient enough. The time complexity of the DP solution is O(nW), where n is the number of items and W is the capacity of the knapsack. That’s okay, but it could be better if W is very large. Space complexity is also O(nW), which is a lot. So, I started thinking about ways to optimize it.
I remembered that we can reduce the space complexity from O(nW) to O(W) by using only two rows of the DP table at a time. We only need the previous row to calculate the current row. So, I modified the code to use this optimization. It made the code a bit more complex, but it reduced the memory usage significantly.
After that, I was pretty happy with the code. It was working correctly, and it was reasonably efficient. I submitted it to whatever online judge thing I was using, and it passed all the test cases. Victory!
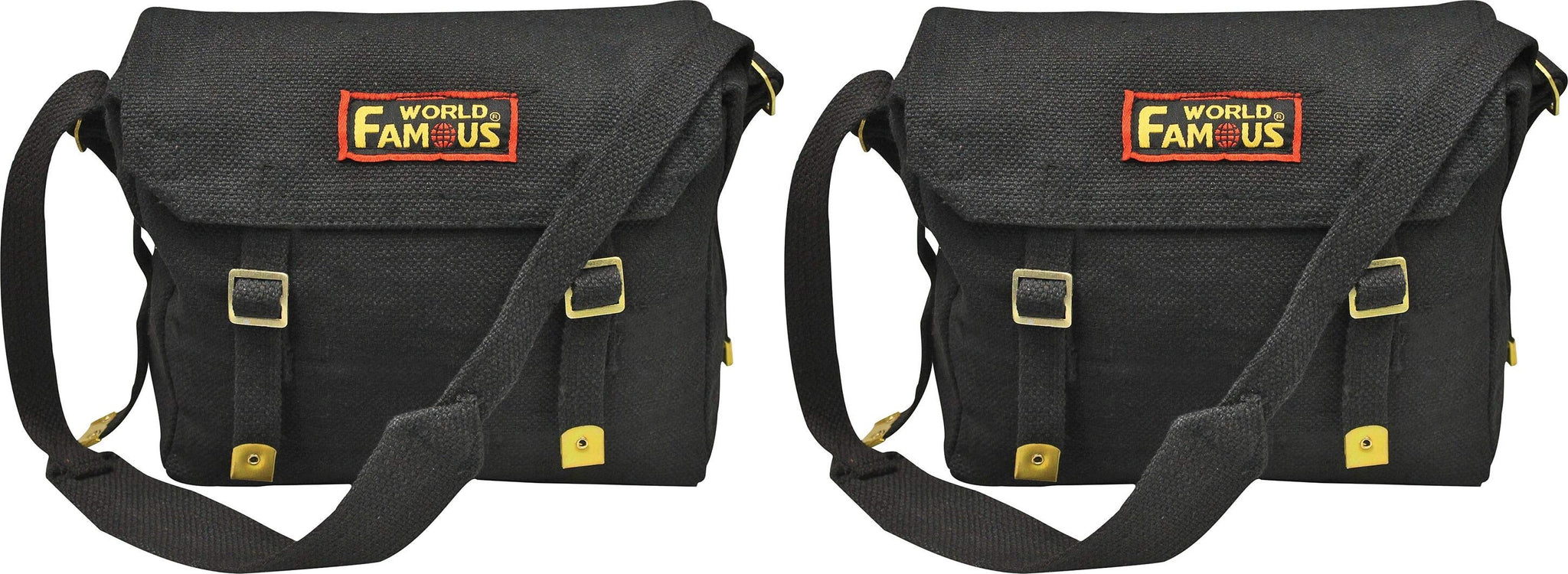
Lessons Learned
- Read the problem description carefully. Make sure you understand all the rules and constraints.
- Start with a simple approach, like brute-force, but be aware of its limitations.
- Dynamic programming is a powerful technique for solving optimization problems.
- Be careful about indexing and off-by-one errors. Debugging DP code can be tricky.
- Optimize your code for both time and space complexity.
So yeah, that was my experience with haversack 12797. It was a fun little challenge, and I learned a few things along the way. Hope this helps someone out there.